In this tutorial, we will learn how to extends multiple class in Java. Is it possible or not in Java and if not possible then how can we accomplish this?
What is Extend in Java?
Extend is one of the keywords in Java which is used for inheritance and indicates that we are inheriting all the properties of the Parent class to the Child class.
The class from which we are inheriting the properties like variables and methods is called as Parent class and the class which is using those properties is called as Child class.
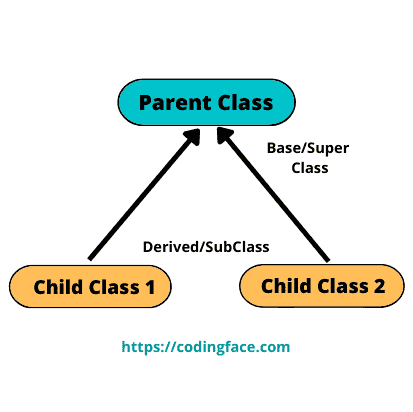
Why do We Need to Extend Multiple Classes?
In many situations, we may need the same properties that we have already developed in other classes. So instead of creating those properties again if we can reuse those properties of other classes, then we can save memory and as well as save our time.
Similarly in Java also we can use other class properties in our class by extending that class.
Ok, Let’s understand through an example.
Suppose, we have a mobile manufacturing company and to develop different types of mobiles, we may need different parts of it like chips, batteries, wires, and many more which are commonly used in most the mobiles. So what can we do is, we will design the same parts with basic functionalities with it and we can use them with different mobiles by modifying them as per our need.
In Java, We can develop one class with some basic functionalities which will be common to other classes and we can extend this class whenever required.
Can We Extend Multiple Classes in Java?
No, We can’t extend multiple classes in Java. As Java doesn’t support Multiple Inheritance, So we can’t extend multiple classes in Java. We can only extend one class and implement multiple Interfaces.
We can declare abstract fields in Interfaces and then we can access them in child classes by using the implement keyword in Java. In this way, we can achieve Multiple Inheritance in Java.
Why It is Not Possible to Extend Multiple Classes in Java?
It is not possible to extend multiple classes in Java because of redundancy. If Java will allow extending of multiple classes, then redundant data may arise.
Though it’s not possible to extend multiple classes. Still, let’s consider like, you have used the same method to perform( ) in two different classes Class A & Class B and you want to access that method in child class. So when you will call that method from a child class, it is not possible to differentiate between which class method you want to call actually. Mostly this problem is similar to Diamond Problem which arises in multiple inheritances.
Later we will try to find the solution for how to extends multiple classe in Java.
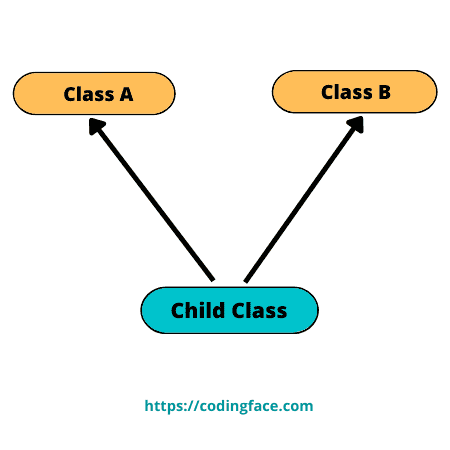
What is Diamond Problem in Java?
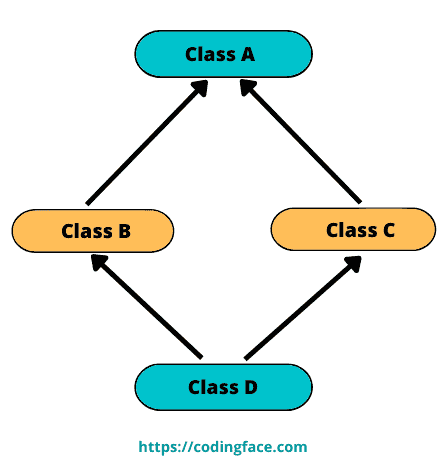
The problem in which one Class D is trying to inherit the same property from two or more than two different classes like Class B and Class C which is again inherited from one parent Class A is called as Diamond Problem in Java.
Suppose we have declared one abstract method perform( ) in abstract Class A and we have two subclasses Class B & Class C extend Class A and also implemented the abstract perform( ) method. Now Class D extends both Class B & Class C (Only, for example, not supported by Java). We have created the obj of Class D and through this obj, we are trying to access the perform( ) method. This will create an ambiguous situation because it will create confusion about which method you are calling the same method we have implemented in both Class B and Class C.
Let’s see the above problem that we have faced through programming for better knowledge so that we will get clear idea about why Java doesn’t support multiple inheritance or why we can’t extend multiple classes in Java.
Diamond Problem Program in Java (Why We Can’t Extend multiple Classes in Java?)
package codingface.java.logical;
public abstract class A {
public abstract void perform();
}
Code language: PHP (php)
package codingface.java.logical;
class B extends A {
public void perform( ) {
System.out.println("Class B perform method");
}
}
Code language: JavaScript (javascript)
package codingface.java.logical;
class C extends A {
public void perform( ) {
System.out.println("Class C perform method");
}
}
Code language: JavaScript (javascript)
package codingface.java.logical;
public class D extends B, C{
public static void main(String[] args) {
D obj = new D();
obj.perform();
}
}
Code language: JavaScript (javascript)
Output
The above class D will give syntax error as well as compile time error if you will forcefully run the above Class D.
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
Syntax error on token "]", :: expected after this token
Syntax error, insert "SimpleName" to complete Type
at codingface.java.logical.D.main(D.java:5)
Code language: CSS (css)
So the above program is the live example of the Diamond Problem in Java and also we have proved that we can’t extend multiple classes in Java.
Let’s see how we can accomplish our goal like how to extends multiple class in Java.
How to Extends Multiple Class in Java?
As we have already discussed that Java doesn’t support multiple inheritance, So we can’t extend multiple classes in Java. We can accomplish our goal of Multiple Inheritance by using the Interface in Java.
Let’s see how we will resolve our query “How to extends multiple class in Java?.
We can extend one class and can implement multiple interfaces in Java which will resolve our problem of creating ambiguity also.
Let’s see the example.
Java Program to Implement Multiple Inheritance
package cf.java.inheritance;
public class Memory {
public void show() {
System.out.println("Memory class show method");
}
}
Code language: PHP (php)
package cf.java.inheritance;
public interface Battery {
public abstract void show();
}
Code language: PHP (php)
package cf.java.inheritance;
public interface Chip {
public abstract void show();
}
Code language: PHP (php)
package cf.java.inheritance;
public class IPhone extends Memory implements Battery, Chip {
@Override
public void show() {
System.out.println("IPhone class show method");
}
public static void main(String[] args) {
IPhone iPhone = new IPhone();
iPhone.show();
Memory memory = new Memory();
memory.show();
}
}
Code language: PHP (php)
Output
IPhone class show method
Memory class show method
Code language: JavaScript (javascript)
Conclusion
In this tutorial, We know about how to extends multiple class in Java.
Though we can’t extends multiple class in Java as Java doesn’t support multiple inheritance, but we can overcome this problem by using interfaces.
Recommended Posts
- Java Program To Print Vowels In A String
- Java Program To Remove Duplicate Words In A String
- Best 2 Ways to Give Tab Space in Java
- How to convert List to Byte Array in Java?
- How To Check String Contains Special Characters In Java?
FAQs
Can We Extend Multiple Classes in Java?
No, We can’t extend multiple classes in Java. We can use interface as an alternatives to accomplish multiple inheritance.
Is It Possible to Implement Multiple Interfaces in Java?
Yes, We can implement multiple interfaces in Java.
Can we Extend Class and Implement Interfaces in Java?
Yes, We can extend one class and implement multiple interfaces simultaneously in Java.
5 thoughts on “How to Extends Multiple Class in Java – Complete Guide 2023”