In this tutorial, We will learn how to reverse an Array in Java by using a simple for loop, by using ArrayList, by using StringBuilder.append( ) and by using ArrayUtils.reverse( ).
What is an Array in Java?
An Array is a collection object which contains multiple elements of the same type. It provides contiguous memory allocation and fixed size of elements. It is also based on indexing which starts from “0”.
Let’s see the below example of an Array with a total of 8 elements where the first index starts from “0” and the last index is “7”. We have differentiated indexes as indices and values as elements. The length of the below Array is 8.
You can also find any particular elements of an Array in Java by using a simple if condition or for loop.
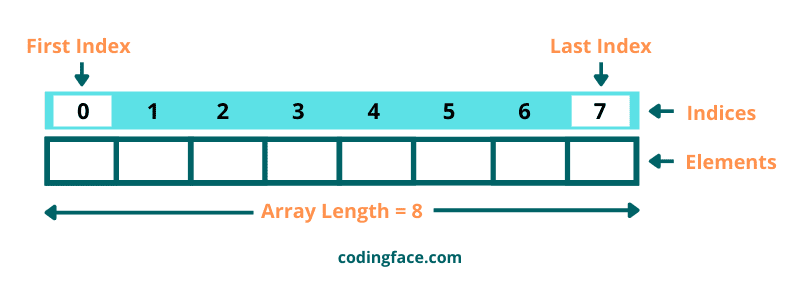
Example of String Array
String[] str = {"Let's", "learn", "Array", "in", "Java"};
Code language: JavaScript (javascript)
Example of Integer Array
int[] num = {45, 25, 65, 8, 47, 5};
Why do we need to reverse an Array in Java?
In the real-time project, we need to access the elements of an Array from the last index to the first index. To retrieve elements of the Array from the last index to the first index, it’s recommended to reverse an Array and then use the elements of that Array.
In most of the MNC’s interviews or coding round, Companies also ask the same type of questions like how to reverse an Array in java without using the predefined methods. So it’s very important to know these concepts before attending the interviews.
Let’s understand how we can reverse an Array in Java.
How to reverse an Array in Java?
We can reverse an Array in Java by using the following methods.
- Reverse an Array by using for loop.
- Reverse an Array by using in Place method (Swapping Method)
- Reverse an Array by using ArrayList and Collections.reverse( ) method
- Reverse an Array by using StringBuilder.append( ) method
- Reverse an Array by using ArrayUtils.reverse( ) method

1. Reverse an Array by using for loop
We can reverse an Array in Java by using a simple for loop. We will iterate through the given Array from the last index to the first index and will store the elements in the new Array one by one.
Once the iteration will be completed, the elements of the Array are also reversed and finally, we can display the reversed Array to the user.
Let’s see the complete program here.
/*
* Java program to Reverse an Array by using for loop.
*/
package programs;
import java.util.Arrays;
public class ReverseAnArrayUsingForLoop {
// revArray() method to reverse a given String
public static void revArray(String givenArray[]) {
// Calculate length of the given String Array
int strLen = givenArray.length;
// Create a new String Array with same length of given Array
String revArray[] = new String[strLen];
int temp = strLen;
/*
* Use for loop to reverse the elements of given Array & store the elements in
* the new Array
*/
for (int i = 0; i < strLen; i++) {
revArray[i] = givenArray[temp - 1];
temp--;
}
// Display the reversed Array
System.out.println("Reversed Array is " + Arrays.deepToString(revArray));
}
public static void main(String[] args) {
// Declare a predefined String Array
String strArray[] = { "Codingface", "is", "a", "Programming", "Tutorial", "Portal" };
// Call revArray( ) method to reverse the given Array
revArray(strArray);
}
}
Code language: JavaScript (javascript)
Output
Reversed Array is [Portal, Tutorial, Programming, a, is, Codingface]
Code language: JavaScript (javascript)
2. Reverse an Array by using in Place method (Swapping Method)
We can reverse an Array in Java by using the in-place method. In this method, we can reverse the Array just by swapping the elements with each other and without using any other predefined methods or another Array. Like, we will swap the last element with the first element within the same Array and 2nd last element with 2nd element, and so on. Finally, the whole Array will be reversed one by one.
Let’s see the complete program here.
/*
* Java program to Reverse an Array by using in Place method (Swapping Method).
*/
package programs;
import java.util.Arrays;
public class ReverseAnArrayUsingInPlaceMethod {
// revArray() method to reverse a given String
public static void revArray(String givenArray[]) {
// Declare a temp variable to store elements
String temp;
// Use for loop to reverse elements of Array one by one
for (int i = 0; i < givenArray.length / 2; i++) {
temp = givenArray[i];
givenArray[i] = givenArray[givenArray.length - i - 1];
givenArray[givenArray.length - i - 1] = temp;
}
// Display the reversed Array
System.out.println("Reversed Array is " + Arrays.toString(givenArray));
}
public static void main(String[] args) {
// Declare a predefined String Array
String strArray[] = { "Codingface", "is", "a", "Programming", "Tutorial", "Portal" };
// Call revArray( ) method to reverse the given Array
revArray(strArray);
}
}
Code language: JavaScript (javascript)
Output
Reversed Array is [Portal, Tutorial, Programming, a, is, Codingface]
Code language: JavaScript (javascript)
3. Reverse an Array by using ArrayList and Collections.reverse() method
We can reverse an Array in Java by using the Collections.reverse( ) method.
As we can not directly reverse an Array by using the Collections.reverse( ) method, So first we will convert the given Array to a List, and then we will reverse the given Array by using the Collections.reverse( ) method.
Let’s see the complete program to understand the above logic.
/*
* Java program to Reverse an Array by using Collections.reverse() method.
*/
package programs;
import java.util.Arrays;
import java.util.Collections;
public class ReverseAnArrayUsingCollectionsReverseFunction {
// revArray() method to reverse a given String
public static void revArray(String givenArray[]) {
// Reverse the given Array by using the Collections.reverse() function
Collections.reverse(Arrays.asList(givenArray));
// Display the reversed Array
System.out.println("Reversed Array is " + Arrays.asList(givenArray));
}
public static void main(String[] args) {
// Declare a predefined String Array
String strArray[] = { "Codingface", "is", "a", "Programming", "Tutorial", "Portal" };
// Call revArray( ) method to reverse the given Array
revArray(strArray);
}
}
Code language: JavaScript (javascript)
Output
Reversed Array is [Portal, Tutorial, Programming, a, is, Codingface]
Code language: JavaScript (javascript)
4. Reverse an Array by using StringBuilder.append( ) method
We can reverse an Array in Java by using the StringBuilder.append( ) method.
First, we will convert the given Array to a StringBuilder by using the append( ) method. Once it will be converted to StringBuilder, we will convert it to an Array by using the String split( ) method.
Let’s see the complete program to understand the above logic.
/*
* Java program to Reverse an Array by using StringBuilder.append() method.
*/
package programs;
import java.util.Arrays;
import java.util.Collections;
public class ReverseAnArrayUsingCollectionsReverseFunction {
// revArray() method to reverse a given String
public static void revArray(String givenArr[]) {
// Declare a new StringBuilder
StringBuilder sb = new StringBuilder();
// Use for loop to convert the elements of Array to StringBuilder
for (int i = givenArr.length; i > 0; i--) {
sb.append(givenArr[i - 1]).append(" ");
}
// Split the StringBuilder var and store it on Array
String[] revArray = givenArr.toString().split(" ");
// Display the reversed Array
System.out.println("Reversed Array is " + Arrays.toString(revArray));
}
public static void main(String[] args) {
// Declare a predefined String Array
String strArray[] = { "Codingface", "is", "a", "Programming", "Tutorial", "Portal" };
// Call revArray( ) method to reverse the given Array
revArray(strArray);
}
}
Code language: JavaScript (javascript)
Output
Reversed Array is [Portal, Tutorial, Programming, a, is, Codingface]
Code language: JavaScript (javascript)
5. Reverse an Array by using ArrayUtils.reverse( ) method
We can reverse an Array in Java by using the ArrayUtils.reverse( ) method.
We can directly reverse the given string by using the ArrayUtils.reverse( ) method. You can just pass the given Array as an argument for the reverse( ) method.
Let’s see the complete program to understand the above logic.
/*
* Java program to Reverse an Array by using ArrayUtils.reverse() method.
*/
package programs;
import java.util.Arrays;
import java.util.Collections;
import org.apache.commons.lang3.ArrayUtils;
public class ReverseAnArrayUsingArrayUtilsReverse {
// revArray() method to reverse a given String
public static void revArray(String givenArray[]) {
// Reverse the given Array by using the ArrayUtils.reverse() function
ArrayUtils.reverse(givenArray);
// Display the reversed Array
System.out.println("Reversed Array is " + Arrays.toString(givenArray));
}
public static void main(String[] args) {
// Declare a predefined String Array
String strArray[] = { "Codingface", "is", "a", "Programming", "Tutorial", "Portal" };
// Call revArray( ) method to reverse the given Array
revArray(strArray);
}
}
Code language: JavaScript (javascript)
Output
Reversed Array is [Portal, Tutorial, Programming, a, is, Codingface]
Code language: JavaScript (javascript)
Conclusion
In this tutorial we have learned how to how to reverse an Array in Java by using different methods like using for loop, in-place method, ArrayList, StringBuilder.append( ) method and ArrayUtils.reverse( ) method.
You can use any of the above approach to reverse your Array. We have taken the examples of only String Arrays where as you can also try for the int Arrays. You just need to replace the String Arrays with int Array without any other changes.
Hope, you can be able to achieve your requirement and liked our tutorial. If our tutorial helped you or if you liked our tutorial, then please share with others.
Thanks & Happy Learning!
Recommended Articles
- 4 Best Ways To Print Array Without Brackets In Java.
- Best 2 Ways To Find The Nth Element In Array Java.
- How To Convert List To Byte Array In Java? – Easy Way
- How To Convert Array To XML In Java – 2 Easy Way
FAQs
How to reverse the order of an array in java?
We can reverse the order of an Array in Java by following methods:
1. Reverse an Array by using a simple for loop through iteration.
2. Reverse an Array by using the in-place method.
3. Reverse an Array by using ArrayList and Collections.reverse( ) method
4. Reverse an Array by using StringBuilder.append( ) method
5. Reverse an Array by using ArrayUtils.reverse( ) method
Is there any predefined methods that we can use to reverse an Array?
Yes, we can use predefined methods like Collections.reverse( ) and ArrayUtils.reverse( ) methods to reverse an Array in Java.
Can we reverse both Integer and String Array?
Yes, We can reverse both Integer and String Arrays in Java. The logic will be same for both of them but we just need to define both Arrays in different programs.